React: A Beginner's Guide
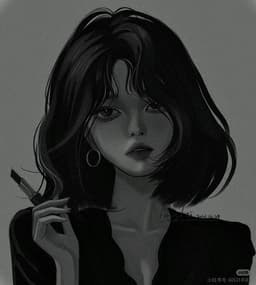
Admin User
January 30, 2025
React is one of the most popular JavaScript libraries for building user interfaces. Whether you're a seasoned developer or just starting out, React's component-based architecture and declarative syntax make it a powerful tool for creating dynamic web applications. In this guide, we'll walk you through the basics of setting up and using React.
Why React?
React, developed by Facebook, is widely used for building single-page applications (SPAs) and reusable UI components. Here are a few reasons why React stands out:
- Component-Based Architecture: Break your UI into reusable components.
- Virtual DOM: Efficiently update and render only the necessary parts of the UI.
- Rich Ecosystem: A vast library of tools, extensions, and community support.
Setting Up Your React Environment
Before diving into React, you'll need to set up your development environment. The easiest way to get started is by using Create React App, a tool that sets up a modern React project with zero configuration.
Step 1: Install Node.js and npm
React requires Node.js and npm (Node Package Manager). If you don’t have them installed, download and install them from nodejs.org.
Step 2: Create a New React Project
Open your terminal and run the following command to create a new React project:
npx create-react-app my-first-react-app
This will create a folder named my-first-react-app
with all the necessary files and dependencies.
Step 3: Start the Development Server
Navigate to your project folder and start the development server:
cd my-first-react-app npm start
Your React app will now be running at http://localhost:3000
.
Understanding the Basics
Components
In React, everything is a component. Components are reusable pieces of code that return HTML (via JSX) to be rendered on the screen. Here's an example of a simple functional component:
import React from 'react'; function Welcome() { return <h1>Hello, React!</h1>; } export default Welcome;
JSX
JSX is a syntax extension for JavaScript that allows you to write HTML-like code in your JavaScript files. It makes your code more readable and easier to write. For example:
const element = <h1>Hello, JSX!</h1>;
Props and State
- Props: Short for "properties," props are used to pass data from one component to another.
- State: State is used to manage data that changes over time within a component.
Here’s an example of using props and state:
import React, { useState } from 'react'; function Greeting({ name }) { const [message, setMessage] = useState(`Hello, ${name}!`); return ( <div> <h1>{message}</h1> <button onClick={() => setMessage('You clicked the button!')}> Click Me </button> </div> ); } export default Greeting;
Styling Your React App
React allows you to style your components using CSS, inline styles, or CSS-in-JS libraries like styled-components. Here’s an example of inline styling:
const styles = { container: { padding: '20px', backgroundColor: '#f0f0f0', }, title: { color: 'blue', }, }; function StyledComponent() { return ( <div style={styles.container}> <h1 style={styles.title}>Styled with Inline CSS</h1> </div> ); }
Deploying Your React App
Once your app is ready, you can deploy it using platforms like Vercel, Netlify, or GitHub Pages. To create a production build, run:
npm run build
This generates an optimized build of your app in the build
folder, which you can then deploy.
Conclusion
React is a powerful and flexible library for building modern web applications. With its component-based architecture and rich ecosystem, it’s no wonder React is a favorite among developers. By following this guide, you’ve taken the first steps toward mastering React. Happy coding!
Code Highlighting with react-syntax-highlighter
To make your code snippets look great, use the react-syntax-highlighter
library. Here's how you can integrate it into your blog:
import { Prism as SyntaxHighlighter } from 'react-syntax-highlighter'; import { atomDark } from 'react-syntax-highlighter/dist/cjs/styles/prism'; const code = `function Welcome() { return <h1>Hello, React!</h1>; }`; function CodeBlock() { return ( <SyntaxHighlighter language="javascript" style={atomDark}> {code} </SyntaxHighlighter> ); }
This will render your code with a sleek, dark theme.
Feel free to customize this post and add your own insights. With react-syntax-highlighter
and remark-gfm
, your blog will look professional and engaging! 🚀